Introduction to Object Oriented Programming (OOP)
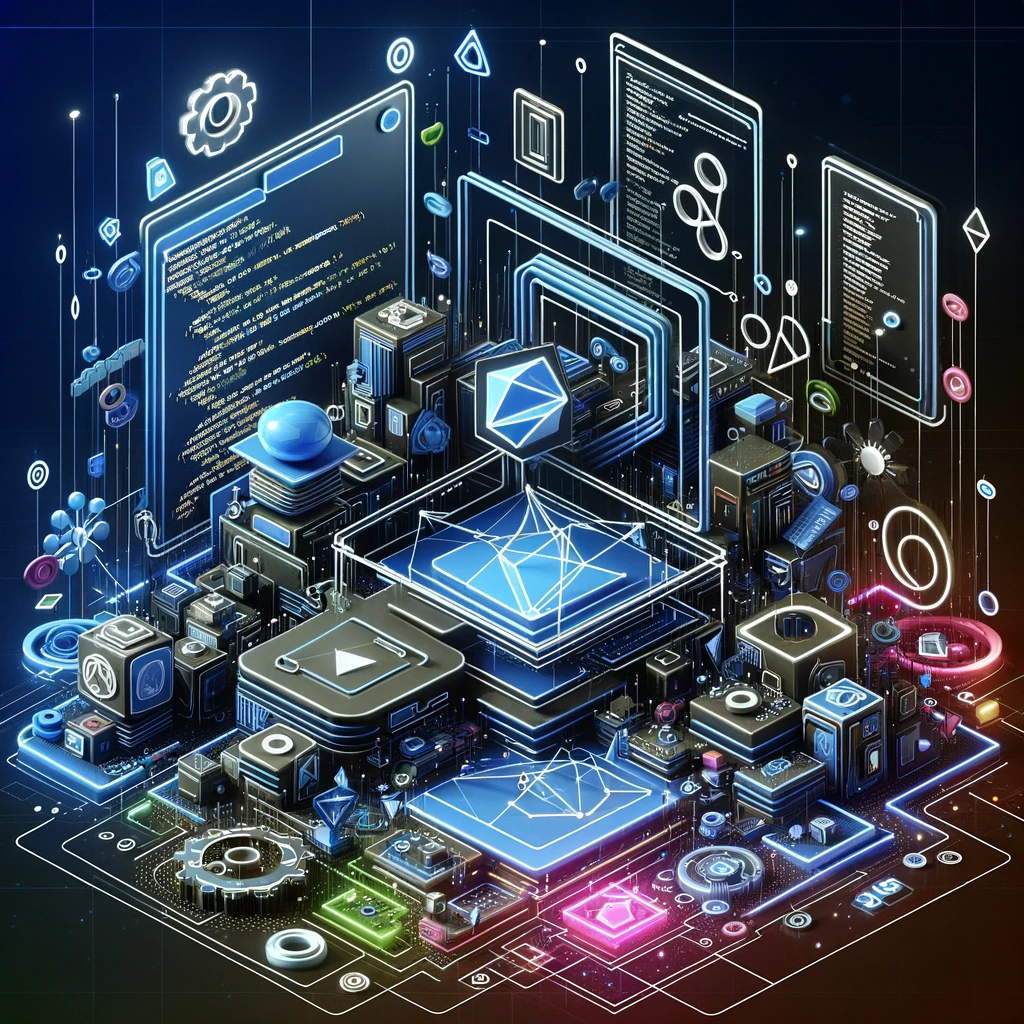
Object-Oriented Programming (OOP) is a paradigm in programming that uses “objects” to design software. Understanding OOP can be made easier through analogies and visuals. Here’s a detailed explanation:
1. Basic Concept of OOP:
OOP revolves around four main concepts: Encapsulation, Abstraction, Inheritance, and Polymorphism.
- Encapsulation: Think of it as a capsule. It’s a way of bundling the data (variables) and methods (functions) that work on the data into a single unit, called an object. For example, a smartphone is an object encapsulating details like its memory size, screen size, and methods like making a call or sending a message.
- Abstraction: This is about hiding the complex reality while exposing only the necessary parts. Like a car, where you don’t need to know the internal workings of the engine to drive it, you only need to know how to operate the steering wheel, pedals, and gears.
- Inheritance: This allows a new class to inherit properties and behavior (methods) from an existing class. Think of it like a child inheriting traits from their parents. A new smartphone model can inherit basic functionalities from a previous model and add new features.
- Polymorphism: This means ‘many forms’. It allows methods to do different things based on the object they are acting upon. Like a single button on a universal remote control, which can control different devices (TV, AC, etc.), a method in OOP can perform different operations depending on the object it is interacting with.
2. Objects and Classes:
- Objects: They are instances of classes. Think of objects as real-world items like your car, a book, or your smartphone.
- Classes: Classes are like blueprints for objects. They define what attributes (like color, size) and methods (like start(), stop()) the object will have.
3. Visual Explanation:
Imagine a class as a blueprint for a house. This blueprint defines the structure of the house like the number of rooms, doors, and windows (attributes), and functionalities like openDoor(), closeWindow() (methods).
When you build a house using this blueprint, you create an object of the class ‘House’. Every house built using this blueprint (class) will have the same structure and functionalities, but each house (object) can have different occupants or different colors.
4. Advantages of OOP:
- Modularity: Easy to understand, maintain, and modify.
- Reusability: Classes can be reused in different programs.
- Scalability: Can easily scale up with the addition of new objects and classes.
Suggested Links for Further Reading:
- Object-Oriented Programming in Python: A beginner-friendly guide to understanding OOP in Python.
- OOP Concepts for Beginners: What is Abstraction?: A detailed explanation of abstraction in OOP.
- Java Inheritance and Polymorphism: A comprehensive guide on inheritance and polymorphism in Java.
- Encapsulation in C#: A focused tutorial on encapsulation using C#.
These resources should give you a well-rounded understanding of the principles of OOP and how they are implemented in various programming languages.